React Audio Player Implementation
Learn to implement Audio Player in React without third-party packages.
Hello my gorgeous friend, ever wondered how music is being added in a react application? In this tutorial, you will learn how to implement an audio player in a react application.
Table of Contents
- What is an audio player
- Adding Audio Player in React
- Examples
- Conclusion
- Resources
What is an audio player
The audio player is a technology that allows you to listen to audio files such as Mp3 and Wav audio formats, the audio player we are going to look into in this tutorial is the web audio player, which allows you to add an audio player to your website for people to listen and dance to ๐.
Adding Audio Player in React
Audio players can be added or implemented in a react component using the HTML audio
element.
Simple right? I know ๐.
The audio
HTML tag makes it possible to play sounds directly from web applications using the below syntax.
<audio src="" controls/>
- The
src
attribute is the path to the audio you want to import. - The
controls
attribute enables the audio player control features such as theplay button
,pause button
,volume control bar
,fast forward button
, and theback forward button
buttons.
Examples
In this section I will show you how to import the audio
file in your react application, there are two ways to achieve this, the first is through the import
method and the other is the require
method.
The Import Method
The import method is part of the JavaScript ES6 features, a method of importing resources such as files, images, and css in JavaScript, you can read more about it here.
The code below demonstrates how to import an audio file using the import method.
import audioSong from "./path-to-audio/lofi-song.jpg";
class AudioPlayer extends React.Component {
render() {
return (
<React.Fragment>
<h1>Audio Player</h1>
<audio src={audioSong} controls />
</React.Fragment>
);
}
}
ReactDOM.render(<AudioPlayer />, document.getElementById('root'));
The audio file is imported using the ES6 method and the import name is passed into the audio tag src
attribute.
import audioSong from "./path-to-audio/lofi-song.jpg";
The Require Method
Another method of importing resources in JavaScript is through the require()
method, a JavaScript file
and module
loader which can be used in adding resources such as audio files as demonstrated below.
class AudioPlayer extends React.Component {
render() {
return (
<React.Fragment>
<h1>Audio Player</h1>
<audio src={require("./path-to-audio/lofi-song.jpg").default} />
</React.Fragment>
);
}
}
ReactDOM.render(<AudioPlayer />, document.getElementById('root'));
In the case of the require()
method, the audio file is imported directly in the src
attribute of the audio
HTML tag through the require()
method.
<audio src={require("./path-to-audio/lofi-song.jpg").default} />
Note: You need to add the .default
to the require() method to return the actual path of the audio file.
Try it yourself result
The final output of the above examples will produce a music player to the browser as shown below.
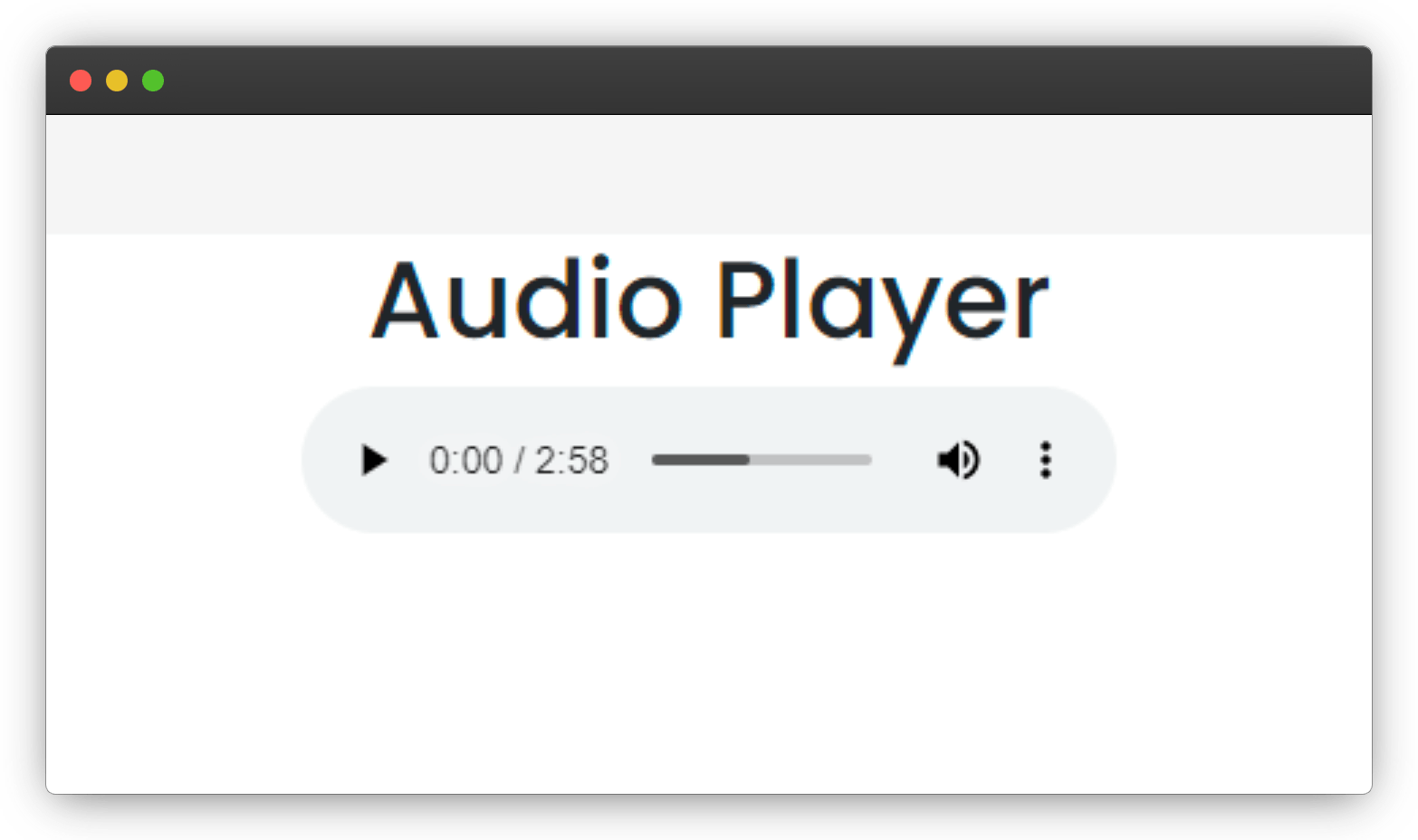
Conclusion
I hope you have learned from this tutorial article how you can implement an audio player in your React Application, this can be useful for adding a music player for your website user to interact with.
Resources
You might want to learn more about the audio player and other ways to implement it in a react application, below are links to useful resources that will be of help.
Wow, what a journey, I am glad you made it to the end of this article, if you enjoyed it and found the content useful, then you shouldn't miss the next one, you can subscribe to my newsletter to get notified of my upcoming contents and articles.
You can subscribe to my newsletter here
I will also like to connect with you, let's connect on:
Wishing you a productive day ahead. Bye Bye ๐โโ๏ธ
If you found my content helpful and would want to support my blog, you can support me by buying me a coffee below, my blog lives on coffee ๐.